Creating a cryptocurrency token on Ethereum has become more accessible with modern development tools. This guide walks through the technical process while comparing popular frameworks, complete with a decision-making table for developers.
Prerequisites for Token Development
-
Solidity knowledge: Basic understanding of smart contract programming
-
Development tools: Node.js, npm, and a code editor like VS Code
-
Web3 wallet: MetaMask configured with testnet ETH
-
Blockchain node: Access via services like Moralis Speedy Nodes
Step-by-Step Development Process
1. Define token specifications
Choose between ERC-20 (fungible) or ERC-721 (NFT) standards based on your use case. Decide on:
-
Token name/symbol
-
Total supply
-
Decimal precision
-
Special features (minting/burning capabilities)
2. Set up development environment
# Using Hardhat
mkdir my-token && cd my-token
npm init -y
npm install --save-dev hardhat
npx hardhat
3. Create ERC-20 contract
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
}
This OpenZeppelin-based contract creates a mintable token with initial supply23.
4. Deploy to testnet
// Deployment script
async function main() {
const [deployer] = await ethers.getSigners();
const Token = await ethers.getContractFactory("MyToken");
const token = await Token.deploy(1000000);
await token.deployed();
console.log("Token address:", token.address);
}
Framework Comparison Table
Tool | Learning Curve | Customization | Speed | Ideal Use Case | Cost |
---|---|---|---|---|---|
Hardhat | Moderate | High | Fast | Complex token logic | Free |
Brownie+Moralis | Low | Moderate | Faster | Rapid prototyping | Freemium |
Bitbond Tool | None | Low | Fastest | Simple tokens (no coding) | Network fees |
Key considerations when choosing a framework
-
Hardhat: Full control over contract logic
-
Moralis: Built-in node infrastructure
-
Bitbond: Zero-code solution
Deployment Best Practices
-
Test thoroughly on Goerli testnet before mainnet launch
-
Implement Access Control for minting/burning functions
-
Verify contracts on Etherscan for transparency
-
Consider gas optimization techniques for user-friendly transactions
Developers can create basic tokens in under an hour using modern tools, while complex implementations may require deeper Solidity expertise. The framework choice ultimately depends on project requirements and technical comfort level.
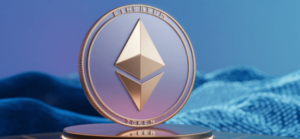
Also Read :